Interaction WolfArray/vectors - Rasterizing
[ ]:
# import _add_path # for debugging purposes only - can be removed in production
from wolfhece import is_enough, __version__
assert is_enough("2.2.7"), "Please update wolfhece to 2.2.7 or later - you have " + __version__
from wolfhece.wolf_array import WolfArray, header_wolf
from wolfhece.PyVertexvectors import Zones, zone, vector
import numpy as np
from packaging import version
Creation of an array
Craate a header
Set shape and resolution
Create an array from the header
[2]:
h = header_wolf()
h.shape = (100, 100)
h.set_resolution(1., 1.)
a = WolfArray(srcheader=h)
Creation of a vector
Square 5m x 5m and 1 attached value named ‘testvalue’.
Create a vector and add vertices from Numpy array
Closed the vector as polygon
Add a value to the vector with the key ‘testvalue’
[3]:
v = vector(fromnumpy= np.asarray([[5.,5.],
[10.,5.],
[10.,10.],
[5.,10.]]))
v.close_force()
v.add_value('testvalue', 5.)
Rasterizing
a bit like RasterIO <https://rasterio.readthedocs.io/en/stable/api/rasterio.rio.rasterize.html#rio-rasterize>
_
[4]:
a.rasterize_vector_valuebyid(v, 'testvalue')
a.plot_matplotlib()
[4]:
(<Figure size 640x480 with 1 Axes>, <Axes: >)
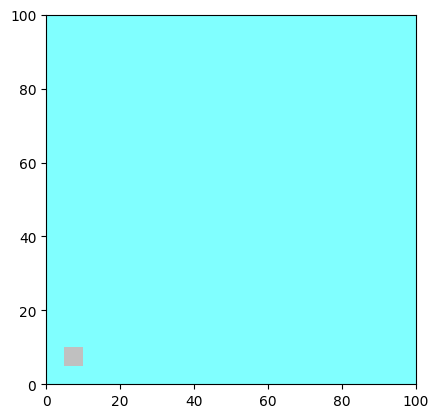
Creation of a zone
[5]:
z = zone()
v2 = vector(fromnumpy= np.asarray([[25.,25.],
[30.,25.],
[50.,35.],
[15.,30.]]))
v2.close_force()
z.add_vector(v, forceparent=True)
z.add_vector(v2, forceparent=True)
z.add_values('testvalue', np.asarray([5., 15.]))
a.rasterize_zone_valuebyid(z, 'testvalue')
fig, ax = a.plot_matplotlib()
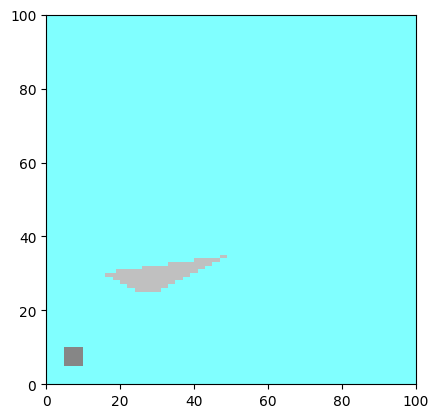
Do not confuse
rasterize_vector_along_grid : routine of header_wolf class –> rasterize a vector along to the grid - very useful for flow discharge computation bsed on arbitrary cross-sections
rasterize_vector_valuebyid : routine of WolfArray class –> fill the grid with the value (by its id) inside the polygon formed by the vector
[5]:
import matplotlib.pyplot as plt
from wolfhece.PyVertex import getRGBfromI, getIfromRGB
v3 = vector(fromnumpy= np.asarray([[25.,25.],
[100.,80.],
[50.,90.],
[60.,10.],
]))
raster_v3 = a.rasterize_vector_along_grid(v3)
fig, ax = plt.subplots()
raster_v3.plot_matplotlib(ax)
v3.myprop.color = getIfromRGB((255,0,0))
v3.plot_matplotlib(ax)
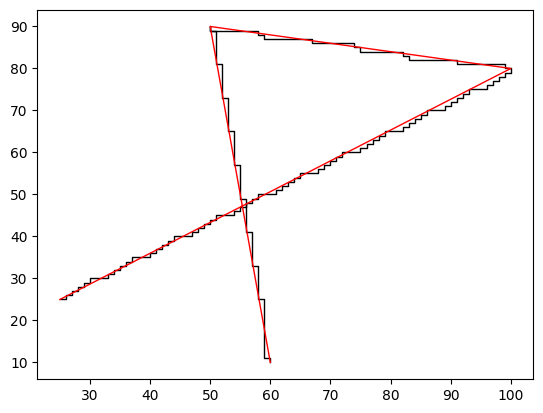