Create an array with a hole at the center
[1]:
from wolfhece.wolf_array import header_wolf, WolfArray
from wolfhece.PyVertexvectors import vector, wolfvertex
import numpy as np
from math import pi, sin, cos
import matplotlib.pyplot as plt
Array part
[2]:
h = header_wolf()
h.set_origin(10.,20.) # to be non-zero
h.set_resolution(.5, .5)
h.shape = 201, 101
wa = WolfArray(srcheader=h)
print(wa)
bounds = wa.get_bounds()
print(bounds)
print(f'The center of the array is at', {(bounds[0][0]+bounds[0][1])/2.}, {(bounds[1][0]+bounds[1][1])/2.})
Shape : 201 x 101
Resolution : 0.5 x 0.5
Spatial extent :
- Origin : (10.0 ; 20.0)
- End : (110.5 ; 70.5)
- Width x Height : 100.5 x 50.5
- Translation : (0.0 ; 0.0)
Null value : 0.0
([10.0, 110.5], [20.0, 70.5])
The center of the array is at {60.25} {45.25}
[3]:
x_center, y_center = wa.ij2xy(101, 51, aswolf=True) # 11, 6 is the center of the array as the array is 201x101
print(f"center = {x_center}, {y_center}")
center = 60.25, 45.25
Vectorial part
[4]:
center_circle = wolfvertex(x_center, y_center)
radius = 5.0
circle = vector(name='circle')
circle.add_vertex([center_circle] *3) # add 3 times the center circle to make a "triangle"
circle.buffer(radius, 5) # create a buffer (-> a cricle) with radius 2.0 and a resolution of 5, 5 segments in a quarter of circle (see https://shapely.readthedocs.io/en/stable/reference/shapely.buffer.html for details)
circle.reset_linestring()
print(circle.nbvertices)
21
Plot just to be sure…
[5]:
fig, ax = plt.subplots()
circle.plot_matplotlib(ax)
ax.set_aspect('equal', adjustable='box')
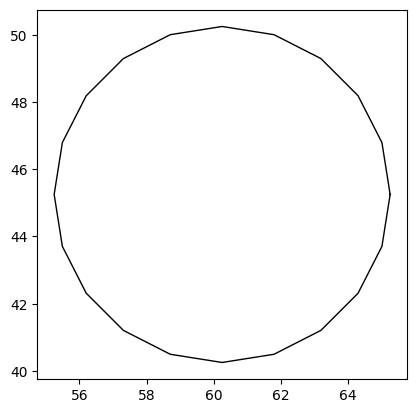
Combine array and vector
[6]:
wa.mask_insidepoly(circle, method='shapely_strict') # mask inside the circle with value 1
wa.nullify_border(1) # nullify 1 cells around the array
Plot just to be sure…
[7]:
fig, ax = wa.plot_matplotlib()
fig.set_size_inches(10, 5)
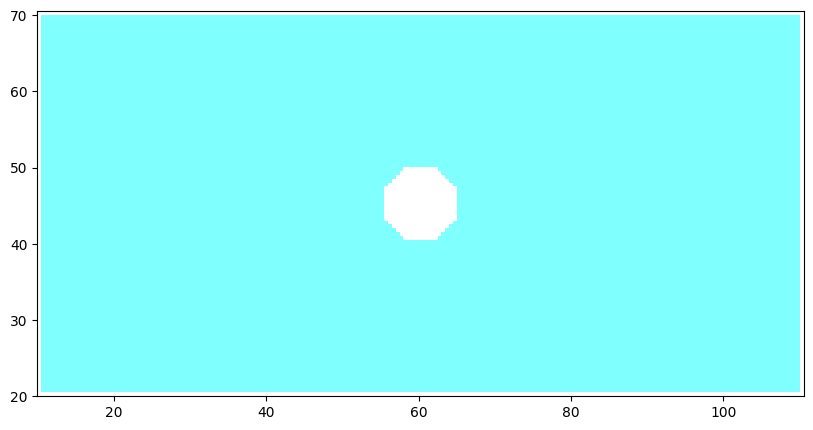
[8]:
fig, ax = wa.plot_matplotlib()
fig.set_size_inches(10, 5)
circle.plot_matplotlib(ax) # plot the circle in red
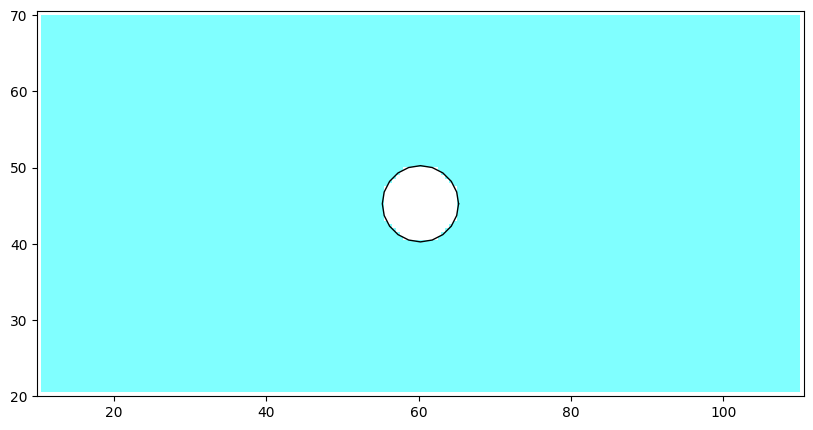
Get the external contour
[9]:
sux, suy, contour, interior = wa.suxsuy_contour()
if interior:
print('There is an interior masked part in the array') # the circle is inside the array
There is an interior masked part in the array
If we plot the contour, the inner part is linked to the outer one.
[55]:
fig, ax = wa.plot_matplotlib()
fig.set_size_inches(10, 5)
contour.plot_matplotlib(ax) # plot the circle in red
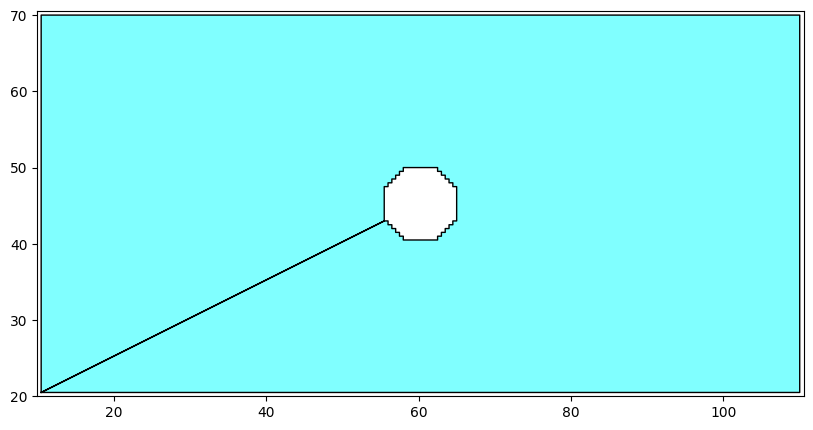
[10]:
print('Number of vertices : ', contour.nbvertices) # Number of vertices in the contour
notused = [curvert for curvert in contour.myvertices if not curvert.in_use]
print('Number of segments not used : ', len(notused)) # Number of vertices/segments not used -- During the meshing process, some segments are not used (links from the external to the internal parts).
# This is normal and helps avoid numerical problems during the process.
#
# Note: An intersection between two segments is not necessarily on these segments... ;-)
Number of vertices : 675
Number of segments not used : 2