Read and manipulate 2D results
In this notebook, we will read and use the results of a 2D CPU simulation.
To do this, it is not necessary to read an entire simulation. However, you must use the Wolfresults_2D object to manipulate the results.
[ ]:
# import _add_path # For debugging purposes - can be removed in production
from wolfhece.wolfresults_2D import Wolfresults_2D
Create the object
We must use the full or relative path to the simulation files, including the generic name of the simulation (without extension).
[2]:
res = Wolfresults_2D(fname = '../_static/2d/test/test')
Number of time steps
[3]:
print("Number of available results : ", res.get_nbresults())
Number of available results : 1001
List of steps
We can get all steps and times.
[4]:
times, steps = res.get_times_steps()
print('Times:', times)
print('Steps:', steps)
assert len(times) == res.get_nbresults()
assert len(steps) == res.get_nbresults()
Times: [ 0. 0.02234928 0.04255178 ... 16.82746 16.844397
16.861334 ]
Steps: [ 0 1 2 ... 998 999 999]
Read one step
To access one simulation step, we can use read_oneresul
. The value used in parameter is 0-based; -1
is the last one.
By default, the view is the water depth but the routine will read h, qx, qy (k and eps if available).
Arrays for each block are stored in a dictionnary myblocks
.
[5]:
res.read_oneresult(-1)
[6]:
res.myblocks.keys()
[6]:
dict_keys(['block1'])
Each block has :
waterdepth
qx
qx
(eps)
These attributes are WolfArray
instances.
[7]:
print(type(res.myblocks['block1'].waterdepth))
print(type(res.myblocks['block1'].qx))
print(type(res.myblocks['block1'].qy))
<class 'wolfhece.wolf_array.WolfArray'>
<class 'wolfhece.wolf_array.WolfArray'>
<class 'wolfhece.wolf_array.WolfArray'>
Access to one specific block
__getitem__
routine is overloaded. You can access one block by its index or its name.
It is not a pointer to the full block result, OneWolfResult
class, but the WolfArray
of the current view.
[16]:
b1 = res[0]
b2 = res['block1']
print(type(b1))
print(type(b2))
<class 'wolfhece.wolf_array.WolfArray'>
<class 'wolfhece.wolf_array.WolfArray'>
Change view type
You can change the current view.
All available type are in views_2D
Enum class.
[19]:
from wolfhece.wolfresults_2D import views_2D
# Print all the available Enum views
print(views_2D.__members__)
res.set_current(views_2D.WATERLEVEL)
{'WATERDEPTH': <views_2D.WATERDEPTH: 'Water depth [m]'>, 'WATERLEVEL': <views_2D.WATERLEVEL: 'Water level [m]'>, 'TOPOGRAPHY': <views_2D.TOPOGRAPHY: 'Bottom level [m]'>, 'QX': <views_2D.QX: 'Discharge X [m2s-1]'>, 'QY': <views_2D.QY: 'Discharge Y [m2s-1]'>, 'QNORM': <views_2D.QNORM: 'Discharge norm [m2s-1]'>, 'UX': <views_2D.UX: 'Velocity X [ms-1]'>, 'UY': <views_2D.UY: 'Velocity Y [ms-1]'>, 'UNORM': <views_2D.UNORM: 'Velocity norm [ms-1]'>, 'HEAD': <views_2D.HEAD: 'Head [m]'>, 'FROUDE': <views_2D.FROUDE: 'Froude [-]'>, 'KINETIC_ENERGY': <views_2D.KINETIC_ENERGY: 'Kinetic energy k'>, 'EPSILON': <views_2D.EPSILON: 'Rate of dissipation e'>, 'TURB_VISC_2D': <views_2D.TURB_VISC_2D: 'Turbulent viscosity 2D'>, 'TURB_VISC_3D': <views_2D.TURB_VISC_3D: 'Turbulent viscosity 3D'>, 'VECTOR_FIELD_Q': <views_2D.VECTOR_FIELD_Q: 'Discharge vector field'>, 'VECTOR_FIELD_U': <views_2D.VECTOR_FIELD_U: 'Velocity vector field'>, 'U_SHEAR': <views_2D.U_SHEAR: 'Shear velocity [ms-1]'>, 'SHIELDS_NUMBER': <views_2D.SHIELDS_NUMBER: 'Shields number - Manning-Strickler'>, 'CRITICAL_DIAMETER_SHIELDS': <views_2D.CRITICAL_DIAMETER_SHIELDS: 'Critical grain diameter - Shields'>, 'CRITICAL_DIAMETER_IZBACH': <views_2D.CRITICAL_DIAMETER_IZBACH: 'Critical grain diameter - Izbach'>, 'CRITICAL_DIAMETER_SUSPENSION_50': <views_2D.CRITICAL_DIAMETER_SUSPENSION_50: 'Critical grain diameter - Suspension 50%'>, 'CRITICAL_DIAMETER_SUSPENSION_100': <views_2D.CRITICAL_DIAMETER_SUSPENSION_100: 'Critical grain diameter - Suspension 100%'>, 'QNORM_FIELD': <views_2D.QNORM_FIELD: 'Q norm + Field'>, 'UNORM_FIELD': <views_2D.UNORM_FIELD: 'U norm + Field'>, 'WL_Q': <views_2D.WL_Q: 'WL + Q'>, 'WD_Q': <views_2D.WD_Q: 'WD + Q'>, 'WL_U': <views_2D.WL_U: 'WL + U'>, 'WD_U': <views_2D.WD_U: 'WD + U'>, 'T_WL_Q': <views_2D.T_WL_Q: 'Top + WL + Q'>, 'T_WD_Q': <views_2D.T_WD_Q: 'Top + WD + Q'>, 'T_WD_U': <views_2D.T_WD_U: 'Top + WD + U'>}
[21]:
fig, ax = res[0].plot_matplotlib()
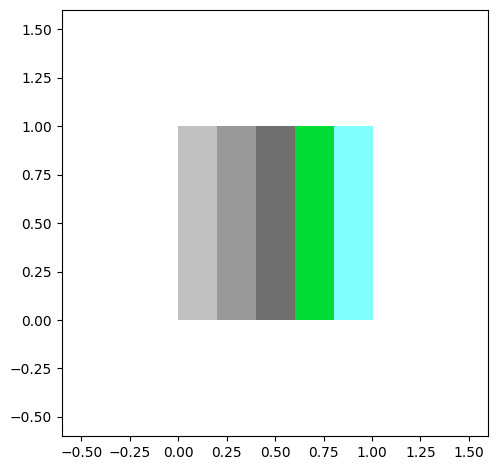
Convert to WolfArray
You can use the as_WolfArray
routine to convert the results to a single array object.
If the simulation contains only one block, the returned type is
WolfArray
.If there are multiple blocks, the returned type is
WolfArrayMB
.
In this example, since the simulation has only one block, a
is an instance of WolfArray
.
[25]:
a = res.as_WolfArray()
print(type(a))
aMB = res.as_WolfArray(force_mb=True)
print(type(aMB))
<class 'wolfhece.wolf_array.WolfArray'>
<class 'wolfhece.wolf_array.WolfArrayMB'>